REST APIs with .NET and C#
ASP.NET makes it easy to build services that reach a broad range of clients, including browsers and mobile devices.
With ASP.NET you use the same framework and patterns to build both web pages and services, side-by-side in the same project.
var app = WebApplication.Create();
app.MapGet("/people", () => new[]
{
new Person("Ana"), new Person("Filipe"), new Person("Emillia")
});
app.Run();
record Person(string Name);
curl https://localhost:5001/people
[{"name":"Ana"},{"name":"Felipe"},{"name":"Emillia"}]
Simple serialization
ASP.NET was designed for modern web experiences. Endpoints automatically serialize your classes to properly formatted JSON out of the box. No special configuration is required. Of course, serialization can be customized for endpoints that have unique requirements.
Authentication and authorization
Secure API endpoints with built-in support for industry standard JSON Web Tokens (JWT). Policy-based authorization gives you the flexibility to define powerful access control rules—all in code.
curl -H "Content-Type: application/json" -X POST -d "{'name':'Ana'}" https://localhost:5001/people/create -i
HTTP/2 202
// MapGroup organizes groups of endpoints under "people"
var group = app.MapGroup("/people");
group.MapGet("/", async (PersonContext db) =>
{
return await db.Person.ToListAsync();
});
group.MapGet("/{id}", async (int id, PersonContext db) =>
{
return await db.Person.FindAsync(id);
});
group.MapPost("/create", async (Person person, PersonContext db) =>
{
db.Person.Add(person);
await db.SaveChangesAsync();
return Results.Created($"/people/{person.Id}", person);
});
app.Run();
Routing alongside your code
ASP.NET lets you define routes and verbs inline with your code, using attributes. Data from the request path, query string, and request body are automatically bound to method parameters.
Designed with security in mind
You don't deploy your apps without security, so why test them without security? ASP.NET provides first class support for HTTPS out of the box. Automatically generate a test certificate and easily import it to enable local HTTPS so you run, and debug, your apps the way they are intended to be... secured.
Fast and scalable
APIs built with ASP.NET Core perform faster than any popular web framework in the independent TechEmpower benchmarks.
Data sourced from official tests available at TechEmpower Round 21.
Easily integrate with Power Platform
Using Power Apps, anyone can build professional-grade business applications with low-code. Extend Power Apps further as a professional developer with custom connectors and logic. Learn how to build these services using OpenAPI-enabled ASP.NET Web APIs and make them available to Power Apps creators.
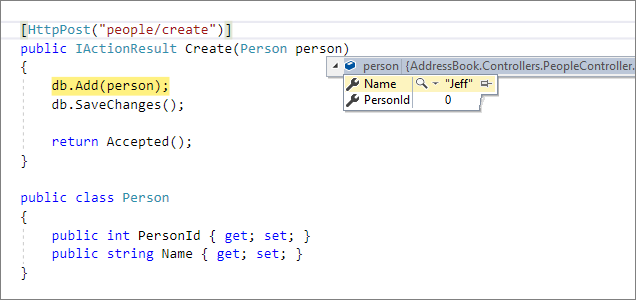
Great tools for any platform
Build, debug, and deploy from any platform to any platform.
Issues in production? Not a problem... simply attach the debugger to your production instance and debug from your laptop!
Start building APIs with ASP.NET Core
Our beginner's guide to building APIs with ASP.NET Core is designed to provide you with the foundation you need to start building APIs with .NET in a collection of short, pragmatic videos.
Ready to get started?
Our step-by-step tutorial will help you get APIs with ASP.NET Core running on your computer.